As a former web-developer myself, I still gravitate back to browser-based UIs. They are so easy to access from anywhere, and are globally available. It’s hard to argue the utility of the amazing web platform. Compose for Web (WASM) is the latest technology in Kotlin Multiplatform and I’m pretty bullish about it.
In this post I’ll take you through what it is and why I think it’s going to be pretty big.
My Past Explorations into Kotlin + Web
I have previously dabbled in Kotlin Multiplatform for JavaScript and Compose HTML in my ShoppingApp project. I find them both very exciting, but I’ve only seen them useful in limited use cases.
I’ve started digging to Compose for Web (WASM), but wanted to give some context to start as there are similarly named things and disambiguation is needed.
Kotlin/JS
This JavaScript (Kotlin/JS) compilation target is here to stay, and is useful not only in the browser, but for backends using Node.js. This technology allows code to be compiled to JavaScript, but doesn’t have any concept of UI itself.
Kotlin/JS is great for complex business logic so it doesn’t have to be re-written and tested in multiple languages.
Kotlin/JS is not so great at size (KBs), and therefore a hard sell to use in Web UIs. I pitched some password validation rule logic to a set of Angular web devs and was told that the JavaScript that was generated was over 10x the size it would be if they wrote it in typescript. That was fair, but I can see the argument tipping the other way if there is really complex business logic. At that point, the consistency and maintainence costs can be more important than page load time (which should get better as time goes on). Note: The reason why the generated JavaScript is so large is because it needs to bring along the Kotlin Standard Library (stdlib), implemented in JS. That is an upfront cost though, so adding additional logic should be fairly linear.
Compose HTML (Previously “Compose for Web”)
Compose HTML allows the compose runtime to render HTML elements. While very cool from a technical standpoint, the marketing behind this has fizzled out (rightfully so) to try and make room for “Compose for Web” (WASM), and create less ambiguity. You can see in this JetBrains blog post first announcing it where it was branded “Compose for Web”, but is now specifically named Compose HTML to disambiguate.
This reminds me of Mosaic, which allows you to leverage the Compose runtime to create terminal apps.
Both are very cool from a technical standpoint, and allow you to leverage the Compose runtime, but you have to bind to platform specific UI elements. Because of this additional work, the developer friction will most likely prevent broader adoption.
Convergence on Compose Multiplatform UI
Jetpack Compose for Android had incredible investment for multiple years and created really solid foundational UI components. The goal is to reuse code that is already written for Jetpack Compose, and bring it to other platforms. That’s why JetBrains uses the same package and classnames in compose-multiplatform. These implementations of compose-multiplatform enable Kotlin Multiplatform UI for Desktop, iOS and now Web (WASM).
These additional (non-Android) multiplatform implementations render to a 2 Dimensional (2D) canvas using SKIA (similar technique used by Flutter). Note: As of very recently, Flutter now leverages the Impeller as the 2D rendering for better performance.
Because a developer can now code against a single set of Compose APIs that can render UIs across multiple platforms, the value is there to start seeing more use cases for Compose Multiplatform UI.
What is Compose for Web (WASM)?
Compose for Web (WASM) leverages Web-Assembly (WASM) to run native binaries in the browser. For browser based UIs, this is a very real future as it’s already supported in Chrome, Safari, Firefox and more. For myself (someone who does Compose UI development for Android), Compose for Web allows me to re-use my existing skills, and create UIs that can be shared via a URL. Not only that, but it is code that will be familiar to other developers on my team, and therefore make it more approachable and have better chance for success.
This Compose for Web technology is current incubating, but is really powerful. Run the samples and check it out, but there are animations and images, and gradients. It’s beautiful.
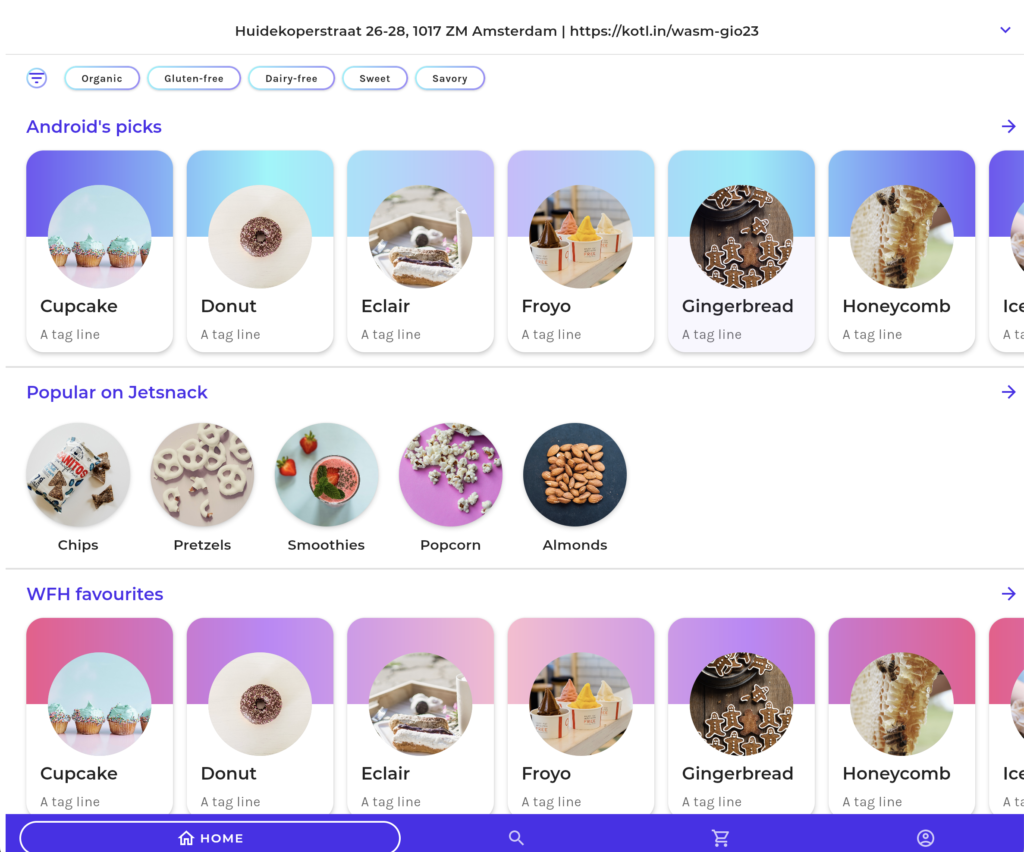
Getting a mobile developer to learn React requires a full paradigm shift and isn’t scalable. The same could be said about shifting to coding in Swift and iOS. Being able to create a consistent set of solutions on a single technology will drive adoption.
Why Compose for Web (WASM)?
I think this will be big for Kotlin developers to get their code running in a browser. I am currently building developer tools for small projects, and making them accessible to everyone in an organization is so much easier to do via a web url instead of having to download an APK and run it.
I have always wanted to share a design system library with others in an organization via a URL. That friction for installing an application is just so cumbersome.
Additionally, we can start to do many test/run cycles in the browser if that test/debug cycle story gets better. I already do that today with Compose for Desktop as it removes the need for a device at all. Android Studio’s Compose previews work great in some use cases, but other times it is nicer to run a bit more code via Compose for Desktop.
With WASM, we will get native performance with this technology, and soon be able to access system level APIs to make very powerful applications.
Next: Under The Surface of Compose for Web (WASM)
I want to dive deeper into Compose for Web (WASM). I’ve been running the samples locally and dived into the generated html, js and wasm files generated by the Compose for Web implementation. As I learn more I’ll share what I learn.
Other related articles I found after writing this:
- The huge potential of Kotlin/Wasm
- https://news.ycombinator.com/item?id=34779746
- https://www.reddit.com/r/Kotlin/comments/12nar6u/on_the_compose_html_rebranding_tldr_everything_is/
To be continued…